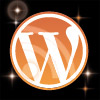
Create simple tooltips with CSS and jQuery – Part 1
CSS tooltips are very popular in modern web design and contrary to popular belief it is really easy to create them, especially with one of the all so popular javascript frameworks.
Before I started to delve deeper into this topic, I thought you have to use at least a plugin, but to get some basic tooltips all you need are about 10 lines of CSS and jQuery Code.
This Tutorial will teach you how to create such tooltips with some basic CSS and jQuery.
Creating jQuery Tooltips: the CSS
First of all take a look of what we are going to create (hover over the link block and don’t care about the background, it’s only for better demonstration of the tooltip transparency)
First of all lets take a look at the structure of this tool tip: A typical link will look something like this:
<a href="#" title="This is a small Tooltip with the Classname 'Tooltip'">Link</a>
We will later use javascript to extract the title and put it into a <p> and a <div> container:
<div class="tooltip"> <p>This is a small Tooltip with the Classname 'Tooltip'</p> </div>
The CSS for our soon to be terrific tooltips looks something like this:
.tooltip{ position:absolute; z-index:999; left:-9999px; background-color:#dedede; padding:5px; border:1px solid #fff; width:250px; } .tooltip p{ margin:0; padding:0; color:#fff; background-color:#222; padding:2px 7px; }
The Position has to be absolute since as soon as our Javascript is enabled it will set the top and left property of the div to display it next to our mouse cursor.
For now we set the left property to -9999px to move the tooltip out of the view port. The rest of the CSS is only for styling purpose.
Creating jQuery Tooltips: the jQuery Code, short and simple
Lets take a look at the whole script, I will give a step by step explanation afterwards:
function simple_tooltip(target_items, name){ $(target_items).each(function(i){ $("body").append("<div class='"+name+"' id='"+name+i+"'><p>"+$(this).attr('title')+"</p></div>"); var my_tooltip = $("#"+name+i); $(this).removeAttr("title").mouseover(function(){ my_tooltip.css({opacity:0.8, display:"none"}).fadeIn(400); }).mousemove(function(kmouse){ my_tooltip.css({left:kmouse.pageX+15, top:kmouse.pageY+15}); }).mouseout(function(){ my_tooltip.fadeOut(400); }); }); } $(document).ready(function(){ simple_tooltip("a","tooltip"); });
This may look intimidating, especially if you are new to jQuery but its really simple. First of all we create the function:
function simple_tooltip(target_items, name){ }
↑ The target item is a variable we will define when calling the script.For example: to append the tooltips to all links in container #maincontent you would enter “#maincontent a”. The name defines the css class we use to style the tooltip. We use variables here for flexibility purpose so you can add diverent tooltips with different stylings.
function simple_tooltip(target_items, name){ $(target_items).each(function(i){ // generates code for each tooltip }); }
↑ This each loop will generate the code for every item that is found by our script. The variable i which we are passing in the function will be automatically incremented by jQuery after each iteration. This way we will be able to give the tooltips unique ids.
function simple_tooltip(target_items, name){ $(target_items).each(function(i){ $("body").append("<div class='"+name+"' id='"+name+i+"'><p>"+$(this).attr('title')+"</p></div>"); }); }
↑ This line creates the html code for each tooltip. They all get the same Classname but different ids. The title is added inside of the div and p container
function simple_tooltip(target_items, name){ $(target_items).each(function(i){ $("body").append("<div class='"+name+"' id='"+name+i+"'><p>"+$(this).attr('title')+"</p></div>"); var my_tooltip = $("#"+name+i); }); }
↑ This line selects the tooltip via jQuery and saves it to a variable for later use
function simple_tooltip(target_items, name){ $(target_items).each(function(i){ $("body").append("<div class='"+name+"' id='"+name+i+"'><p>"+$(this).attr('title')+"</p></div>"); var my_tooltip = $("#"+name+i); $(this).removeAttr("title").mouseover(function(){ }).mousemove(function(kmouse){ }).mouseout(function(){ }); }); }
↑ This is the basic construct of our functions centerpiece. First of all we select the current link with $(this). Then the title attribute is removed since we don’t want to display the “normal” tooltip that every browser displays when hovering over links.
Then we prepare 3 functions:
- The mouseover function is called when you first hover over the link
- The mousemove function is called when we move the mouse while hovering over the link
- The mouseout function is called as soon as the mouse leaves the link
As you can see we pass a parameter in mousemove: this parameter is very important since it stores the position of the mousecursor!
$(this).removeAttr("title").mouseover(function(){ my_tooltip.css({opacity:0.8, display:"none"}).fadeIn(400); }).mousemove(function(kmouse){ my_tooltip.css({left:kmouse.pageX+15, top:kmouse.pageY+15}); }).mouseout(function(){ my_tooltip.fadeOut(400); });
↑ Now we define whats happening when the different functions are called:
On mouseover we set some css values for the tooltip: we define the transparency and set the display to none. Then the div slowly fades in because of the fadeIn call.
On mousemove we constantly set the positioning values left and top to align the tooltip next to the cursor. the X and Y coordinates are called via .pageX and .pageY. We also add a little offset of 15 px so the tooltip is not directly below the cursor
On mouseout we simply call fadeOut to hide the tooltip
$(document).ready(function(){ simple_tooltip("a","tooltip"); });
↑ Last thing we do is: we call the script as soon as the document is loaded. As mentioned earlier parameter 1 is the selector and parameter 2 is the classname of our tooltip. This way you can create multiple designs for your tooltips.
The code we just created can be modified in various ways. I have used a modified version for images in a lately created showcase wordpress theme for Themeforest.net.
Thats is, have fun creating your own sleek tooltips ;)
Update:
Suggested by my readers here is a version of the script that checks if the link has a tittle:
function simple_tooltip(target_items, name){ $(target_items).each(function(i){ $("body").append("<div class='"+name+"' id='"+name+i+"'><p>"+$(this).attr('title')+"</p></div>"); var my_tooltip = $("#"+name+i); if($(this).attr("title") != ""){ // checks if there is a title $(this).removeAttr("title").mouseover(function(){ my_tooltip.css({opacity:0.8, display:"none"}).fadeIn(400); }).mousemove(function(kmouse){ my_tooltip.css({left:kmouse.pageX+15, top:kmouse.pageY+15}); }).mouseout(function(){ my_tooltip.fadeOut(400); }); } }); } $(document).ready(function(){ simple_tooltip("a","tooltip"); });
Update 2:
Here is part 2 of the Tutorial: Create simple tooltips with CSS and jQuery – Part 2: Smart Tooltips

Hey Christian or Kriesi.
Thank your for such a good tutorial!
It’s really nice to know that you can make yourself a tooltip with less css and javascript skills.
I will definitly use it on my website.
Pardon,
But it is not working in firfox3.03.
Works perfect for me in firefox 3.03 for me, both on WinXP and MacOSX :/
This is a pretty nice tooltip script. One thing I didn’t like about it AT FIRST was that I thought I’d have to provide a title attribute for every link on my page or I’d get an empty tooltip box when hovering over a link.
I discovered I could change the script slightly to include a class attribute on the anchor and that would limit the effect to only the ones that I had supplied the class for. The new class was “_tooltip” to keep it separate from the existing “tooltip” class.
Then I took it a step further and styled different tips adding 2 more classes “_tooltip2” and “_tooltip3”.
The only modifications to the script above come at the very end …
$(document).ready(function(){
simple_tooltip(“a._tooltip”,”tooltip”);
simple_tooltip(“a_tooltip2″,”tooltip2”);
simple_tooltip(“a._tooltip3″,”tooltip3”);
});
and of course I have to modify my css accordingly:
.tooltip, .tooltip2, .tooltip3 {
position:absolute;
z-index:999;
left:-9999px;
background-color: #dedede;
padding:5px;
border:1px solid #fff;
width:150px;
}
.tooltip p, .tooltip2 p, .tooltip3 p {
margin:0;
padding:0;
color:#fff;
padding:2px 7px;
}
.tooltip p {
background-color:#C16C29;
}
.tooltip3 p {
background-color: green;
}
you are using the script exactly the way I thought it should be used =)
You can of course add an if statement and check i a title is available at the beggining of the script
Something like this:
if($(this).attr(‘title’) == “”){return false;}
Hi, great script!
I’m quite the novice to jQuery (and js in general, to be honest), but I already love it big time. The only problem is that because of that, I can find it a bit tricky to customize scripts.
I liked the idea of the added statement checking for titles first, but I can’t seem to make it work. Is it really just to add it to the beginning of the script? I’ve done that, and it gives me nothing…
the one line I threw in was just an idea (that doesn’t work all too good, i must admit^^), I have updated the tutorial, you can find the whole updated script at the end.
It now checks if a title is set and should work without problems.
I don’t know if I’m doing something wrong, but even with that new script, there are tooltips on all links, even those without titles.
This should work as you can see here:
https://kriesi.at/wp-content/extra_data/tooltip_tutorial/step3.html
If you are working on a live site maybe I can help you if tell me the url.
The url is http://ricochet.blogg.se/
the js is at http://templates.gridpool.se/sofies/js/tooltip3.js
guess this could be because you are using an old version of jquery. I have only tested my script with v.1.2.6, You are using 1.2.2 which is pretty old =)
Maybe you should consider updating it =)
Another option i guess would be to change the if statement to:
if($(this).attr(“title”) != “” || $(this).attr(“title”) != “undefined”){ etc
Okay, thanks I’ll try if updating jQuery helps :)
I think I found the problem! I had two conflicting jQuery files, one that was 1.2.6, and one that was 1.2.2
arr, sloppy coding on my side, I’m glad everything works now now. Thanks for your help!
great post ! thanks !
(Y)++
Hey,
I used it on my site (just a fun project to learn a little bit xhtml and css) and I’m searching a possibility to slide the tooltips to the left side if they would otherwise showed outside of the browser-window (for example with the resulution of 1024×768 the tooltips at the right side are not showed inside the window).
Do you have an idea?
Already did it, cause I needed the same =)
I am going to release Part 2 of this tutorial during the upcoming days ;)
Perfect :D thank you very much!
I’m currently trying to throw this tooltip into my site but can’t seem to get it working at all. Is there a zip file or anything that you can download full functioning files. I’ve tried looking at the source code but still doesn’t seem to work.
Thank you.
I tried to see it work on different sites named here by your fans. on FF 3.0.5 I use now it is not always running. Same for other browsers although it works nice on th3.d34th’s website.
Anyway I like the tooltip alot. Best of wishes to you and keep up the nice work ;)