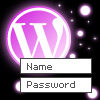
Admin login on your front page
Last week I showed you how to create a menu which reveals itself only to logged in users, utilizing the WordPress function current_user_can(). Today we will take this one step further and create a Login form for your users, which can be placed in your sidebar, footer or anywhere else on your page.
The final result would look something like this on a WordPress default installation:
So what we want to display is:
- An input field for user name
- An input field for password
- A checkbox for remembering the user
- A hidden field which tells WordPress where to redirect the User after login took place
- A Submit Button
- 2 Links: One for password recovery, one for new registration
Since we want to make the form work in every template we will make use of the function get_option(‘home’) which gets the the full path to your WordPress installation.
<h3>Login</h3> <form action="<?php echo get_option('home'); ?>/wp-login.php" method="post"> <p><label for="log">User</label><input type="text" name="log" id="log" value="<?php echo wp_specialchars(stripslashes($user_login), 1) ?>" size="20" /> </p> <p><label for="pwd">Password</label><input type="password" name="pwd" id="pwd" size="20" /></p> <p><input type="submit" name="submit" value="Send" class="button" /></p> <p> <label for="rememberme"><input name="rememberme" id="rememberme" type="checkbox" checked="checked" value="forever" /> Remember me</label> <input type="hidden" name="redirect_to" value="<?php echo $_SERVER['REQUEST_URI']; ?>" /> </p> </form> &s <a href="<?php echo get_option('home'); ?>/wp-register.php">Register</a> <a href="<?php echo get_option('home'); ?>/wp-login.php?action=lostpassword">Recover password</a>
Into the hidden field, we put the URL of the page the user used to log in, so he is redirected to this page again after authentication.
Since we only want to show this code if the user is not already logged in, we use the function current_user_can() , with a parameter of level_0. (in case you dont understand what that means check my last tutorial about current_user_can)
<?php if (!(current_user_can('level_0'))){ ?> <h3>Login</h3> <form code from above etc> <?php } else { /*next to come*/}?>
The next thing to do is writing the code for logged in users. For this purpose I just take a slightly modified version of last weeks tutorial (mentioned above) and put it in the else condition. Here is how the whole thing looks like, just in case you only want to copy/paste it ;)
<?php if (!(current_user_can('level_0'))){ ?> <h3>Login</h3> <form action="<?php echo get_option('home'); ?>/wp-login.php" method="post"> <p><label for="log">User</label><input type="text" name="log" id="log" value="<?php echo wp_specialchars(stripslashes($user_login), 1) ?>" size="20" /> </p> <p><label for="pwd">Password</label><input type="password" name="pwd" id="pwd" size="20" /></p> <p><input type="submit" name="submit" value="Send" class="button" /></p> <p> <label for="rememberme"><input name="rememberme" id="rememberme" type="checkbox" checked="checked" value="forever" /> Remember me</label> <input type="hidden" name="redirect_to" value="<?php echo $_SERVER['REQUEST_URI']; ?>" /> </p> </form> <a href="<?php echo get_option('home'); ?>/wp-register.php">Register</a> <a href="<?php echo get_option('home'); ?>/wp-login.php?action=lostpassword">Recover password</a> <?php } else { ?> <ul class="admin_box"> <li><a href="<?php echo get_option('home'); ?>/wp-admin/">Dashboard</a></li> <li><a href="<?php echo get_option('home'); ?>/wp-admin/post-new.php">Write new Post</a></li> <li><a href="<?php echo get_option('home'); ?>/wp-admin/page-new.php">Write new Page</a></li> <li><a href="<?php echo get_option('home'); ?>/wp-login.php?action=logout&redirect_to=<?php echo urlencode($_SERVER['REQUEST_URI']) ?>">Log out</a></li> </ul> <?php }?>
Voila, your front page login is ready to use ;)
Update for wordpess 2.7
If your are using wordpress 2.7 you have to exchange the old logout link with the new version:
<a href="<?php echo wp_logout_url(urlencode($_SERVER['REQUEST_URI'])); ?>logout</a>

Thanks a lot for this.. i had been trying to get this done, but my knowledge of php really hit the maximum.. and i couldn’t get it done. Thanks!
I’m glad I could help you ;)
Hi Kriesi
Thanks for this tutorial. I’ve been trying to implement this on a new blog and have been stumped. Your solution is the closest so far, but it’s breaking the page and not letting me login.
You can see it here:
http://awakeningtogreatness.com/members/signup/
I’m probably doing something obvious (to you). If you have a chance to take a look, I’d most definitely appreciate it.
Thanks
Hi Edward,
Seems some quotation marks got messed up during the copy/paste process.
Your page breaks at the hidden input field which defines the redirect. The second quotation mark of the value attribute doesn’t close the attribute.
Should work if you retype it by hand.
Awesome. That was the problem. In fact, I had to go in and change about half of the quotes and apostrophes. Not sure why some of them pasted correctly and others pasted as fancy characters. But anyway, it works now.
Great tip. I’ve stumbled this post.
Thanks.
Hi Kriesi,
Thanks for this great tutorial.
I wonder if you have a solution for adding an “Add to Favorite” link to every post, where registered users are allowed to add their favorite posts to their profile or something.
If you know a plug-in doing that let me know.
Thanks a lot, your feedback is appreciated.
Thanks for this coding. Worked for what I needed to do. Tweaked it so that I have different menus than regular users. Thanks for this took me hours to find.
You are welcome ;)
Hi,
That’s one great tutorial. Thanx.
Btw. I think you could make it better by putting everything in one file for download. It’s a bit confusing to copy-paste the code at the moment. Especially for people with limited knowledge like me.
Hey dude.
Thank a lot..!
I had been finding this for a long time.
hi
i need help. i have a site which has a user login. i want to link that with my word press login ie have common login. plz help
thanx in advance
vasu
Thanks for this, will try it out. This is the most comprehensive tutorial I’ve found on this so far. You’re a rock star!
ÑпаÑибо, реальный Ñайтик.
i’ve been crawling your site :) and find it very useful..
Thank u for being generous.. your my favorite!
btw.. what is the plugin u use for the main menus? so fabulous!
never mind! it’s on your tutorial! you’re rock!
Nie wiem czy juz ktos poruszal ten temat, ale na stronie http://www.dopalacze.com mozna kupic legalne dragi (nie zawierajace zabronionych w Polsce substancji) – i sa lepsze od zabronionych srodkow – lepiej wala i dluzej trzymaja – wystarczy poczytac recenzje tabletek diablo.
Bylbym wdzieczny za wpisanie mojego maila: dekadent30@gmail.com jako osoby polecajacej przy rejestracji do sklepu
pozdrawiam
Ciekawa strona, bede ja odwiedzal czesciej, pozdro
Thank you so much, I was having a lot of trouble trying to figure this one out..!!
I like it! But how best to include this into a design? Should I create a widget from it? Or should the code go straight into the template files? I’m just thinking about an easy way to maintain this on the long term. cheers!
Thanks a lot!!!!!!!! This will be helpful lot coz im going to create a website using wordpress
This works until I log in, then it is saying I’m looking for something that isn’t there!
Hi,
err.. i might sound stupid asking this question, actually where shoud i implement this? on every template i use or as a widget or something similar. I’m using pluggin that would execute php so i could use this as widget i guess.
I’m not much familiar with WP templates so i’m not sure where to put the code, that’s the reason i’m asking this.
Thanks. and love your stuff. nice site.
BTW i just found a glitch in your “log Out” link. this is since word press 2.7, they use a few addition chars to log out a person. may be make sure of security and make sure its the same person they’re loggin out. here’s the modified version of the log out link.
FIND:
<a href=”/wp-login.php?action=logout&redirect_to=”>Log out
REPLACE WITH:
<a href=””>Log out
This method is for WP 2.7+
Nice. Thanks. I think you’re missing the closing ‘”>’ for the logout link snippet for WordPress 2.7
<a href="logout
Sir, you forgot to include this before the text “logout”
?>">logout
double quot + less than sign
Nice guide