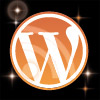
Create simple tooltips with CSS and jQuery – Part 1
CSS tooltips are very popular in modern web design and contrary to popular belief it is really easy to create them, especially with one of the all so popular javascript frameworks.
Before I started to delve deeper into this topic, I thought you have to use at least a plugin, but to get some basic tooltips all you need are about 10 lines of CSS and jQuery Code.
This Tutorial will teach you how to create such tooltips with some basic CSS and jQuery.
Creating jQuery Tooltips: the CSS
First of all take a look of what we are going to create (hover over the link block and don’t care about the background, it’s only for better demonstration of the tooltip transparency)
First of all lets take a look at the structure of this tool tip: A typical link will look something like this:
<a href="#" title="This is a small Tooltip with the Classname 'Tooltip'">Link</a>
We will later use javascript to extract the title and put it into a <p> and a <div> container:
<div class="tooltip"> <p>This is a small Tooltip with the Classname 'Tooltip'</p> </div>
The CSS for our soon to be terrific tooltips looks something like this:
.tooltip{ position:absolute; z-index:999; left:-9999px; background-color:#dedede; padding:5px; border:1px solid #fff; width:250px; } .tooltip p{ margin:0; padding:0; color:#fff; background-color:#222; padding:2px 7px; }
The Position has to be absolute since as soon as our Javascript is enabled it will set the top and left property of the div to display it next to our mouse cursor.
For now we set the left property to -9999px to move the tooltip out of the view port. The rest of the CSS is only for styling purpose.
Creating jQuery Tooltips: the jQuery Code, short and simple
Lets take a look at the whole script, I will give a step by step explanation afterwards:
function simple_tooltip(target_items, name){ $(target_items).each(function(i){ $("body").append("<div class='"+name+"' id='"+name+i+"'><p>"+$(this).attr('title')+"</p></div>"); var my_tooltip = $("#"+name+i); $(this).removeAttr("title").mouseover(function(){ my_tooltip.css({opacity:0.8, display:"none"}).fadeIn(400); }).mousemove(function(kmouse){ my_tooltip.css({left:kmouse.pageX+15, top:kmouse.pageY+15}); }).mouseout(function(){ my_tooltip.fadeOut(400); }); }); } $(document).ready(function(){ simple_tooltip("a","tooltip"); });
This may look intimidating, especially if you are new to jQuery but its really simple. First of all we create the function:
function simple_tooltip(target_items, name){ }
↑ The target item is a variable we will define when calling the script.For example: to append the tooltips to all links in container #maincontent you would enter “#maincontent a”. The name defines the css class we use to style the tooltip. We use variables here for flexibility purpose so you can add diverent tooltips with different stylings.
function simple_tooltip(target_items, name){ $(target_items).each(function(i){ // generates code for each tooltip }); }
↑ This each loop will generate the code for every item that is found by our script. The variable i which we are passing in the function will be automatically incremented by jQuery after each iteration. This way we will be able to give the tooltips unique ids.
function simple_tooltip(target_items, name){ $(target_items).each(function(i){ $("body").append("<div class='"+name+"' id='"+name+i+"'><p>"+$(this).attr('title')+"</p></div>"); }); }
↑ This line creates the html code for each tooltip. They all get the same Classname but different ids. The title is added inside of the div and p container
function simple_tooltip(target_items, name){ $(target_items).each(function(i){ $("body").append("<div class='"+name+"' id='"+name+i+"'><p>"+$(this).attr('title')+"</p></div>"); var my_tooltip = $("#"+name+i); }); }
↑ This line selects the tooltip via jQuery and saves it to a variable for later use
function simple_tooltip(target_items, name){ $(target_items).each(function(i){ $("body").append("<div class='"+name+"' id='"+name+i+"'><p>"+$(this).attr('title')+"</p></div>"); var my_tooltip = $("#"+name+i); $(this).removeAttr("title").mouseover(function(){ }).mousemove(function(kmouse){ }).mouseout(function(){ }); }); }
↑ This is the basic construct of our functions centerpiece. First of all we select the current link with $(this). Then the title attribute is removed since we don’t want to display the “normal” tooltip that every browser displays when hovering over links.
Then we prepare 3 functions:
- The mouseover function is called when you first hover over the link
- The mousemove function is called when we move the mouse while hovering over the link
- The mouseout function is called as soon as the mouse leaves the link
As you can see we pass a parameter in mousemove: this parameter is very important since it stores the position of the mousecursor!
$(this).removeAttr("title").mouseover(function(){ my_tooltip.css({opacity:0.8, display:"none"}).fadeIn(400); }).mousemove(function(kmouse){ my_tooltip.css({left:kmouse.pageX+15, top:kmouse.pageY+15}); }).mouseout(function(){ my_tooltip.fadeOut(400); });
↑ Now we define whats happening when the different functions are called:
On mouseover we set some css values for the tooltip: we define the transparency and set the display to none. Then the div slowly fades in because of the fadeIn call.
On mousemove we constantly set the positioning values left and top to align the tooltip next to the cursor. the X and Y coordinates are called via .pageX and .pageY. We also add a little offset of 15 px so the tooltip is not directly below the cursor
On mouseout we simply call fadeOut to hide the tooltip
$(document).ready(function(){ simple_tooltip("a","tooltip"); });
↑ Last thing we do is: we call the script as soon as the document is loaded. As mentioned earlier parameter 1 is the selector and parameter 2 is the classname of our tooltip. This way you can create multiple designs for your tooltips.
The code we just created can be modified in various ways. I have used a modified version for images in a lately created showcase wordpress theme for Themeforest.net.
Thats is, have fun creating your own sleek tooltips ;)
Update:
Suggested by my readers here is a version of the script that checks if the link has a tittle:
function simple_tooltip(target_items, name){ $(target_items).each(function(i){ $("body").append("<div class='"+name+"' id='"+name+i+"'><p>"+$(this).attr('title')+"</p></div>"); var my_tooltip = $("#"+name+i); if($(this).attr("title") != ""){ // checks if there is a title $(this).removeAttr("title").mouseover(function(){ my_tooltip.css({opacity:0.8, display:"none"}).fadeIn(400); }).mousemove(function(kmouse){ my_tooltip.css({left:kmouse.pageX+15, top:kmouse.pageY+15}); }).mouseout(function(){ my_tooltip.fadeOut(400); }); } }); } $(document).ready(function(){ simple_tooltip("a","tooltip"); });
Update 2:
Here is part 2 of the Tutorial: Create simple tooltips with CSS and jQuery – Part 2: Smart Tooltips

Nice tutorial (y)
i am yet to move to part 2 but that looks easy enough to do ;)
anyway, i have altered some code for easier customisation and to stop some of the reported flickering.
firstly, i have added the name attribute to the anchor tags which i use to reference what css class to use,
$(“body”).append(“”+$(this).attr(‘title’)+””);
so rather than putting several
simple_tooltip(“a._tooltip”,”tooltip”);
simple_tooltip(“a_tooltip2″,”tooltip2”);
simple_tooltip(“a._tooltip3″,”tooltip3”);
it uses the name tag of the anchor to locate the right css :)
(it could do with a default adding, but i havn’t got round to that.)
secondly, rather than using the nasty fadeIn, fadeOuts i have altered them to the following.
$(this).removeAttr(“title”).mouseover(function(){
my_tooltip.animate({opacity:0.8},{queue:false});
})
and
.mouseout(function(){
my_tooltip.animate({opacity:0},{duration:400,queue:false});
});
they do the same trick as the fade ins and outs, but you dont get the nasty flickering and bugging.
peace out x
Hi,that’s a damn good checklist!any chance you could make it into a pdf for us all?
Hi Kriesi,
Great theme. I’ve been trying for awhile to integrate an image tooltip into my gallery – but I haven’t been able to do so in WordPress. I’m trying to use the function, which looks like this:
<a href="” class=”tooltip”>
I haven’t succeeded. Any suggestions?
Thanks!
The php didn’t come through on my comment…sorry about that. And I was referring to your gallery theme which uses your modified tooltip.
Thanks for any advice.
Brilliant blog and really can assist with comprehending the issue much better.
Got a problem with Create simple tooltips with CSS and jQuery part 1
i am try to apply tooltips to only one section of my site, but instead its doing it for every link i have with a title attribute,
i have my main container then my wrapper and containers within this section.
how do i apply tooltips to only one one of this may containers which i have within my wrapper
anyone got any ideas
cheers
rejplica
@Anil
All you have to do is put a class on the links you want the tooltips to appear on
example:
then just add it to the fuction
$(document).ready(function(){
simple_tooltip(“a.toolt”,”tooltip”);
});
comment removed my code
a class = ” toolt “
Very nice article and a great read for beginners.
I like your article very much. With your rich knowledge, we can learn more from your wonderful post.
This is exactly what i was looking for great!… just one thing….. i am fine with the css but how to I get the jquery script above into my wordpress theme (a step by step would be good)
i know all about ftp and stuff i just am clueless with how to get the jquery bit done.
any help would be greatly appreciated!